Yolxander Jaca
Senior Full Stack Developer
Crafting robust web applications with Laravel, PHP, and modern web technologies. With 6+ years of experience, I bring ideas to life through clean, efficient, and scalable code.
About Me
With over 7 years of experience in web development, I've honed my skills in building robust and scalable applications using Laravel and related technologies. My expertise spans full-stack development, with a strong focus on backend architecture, API design, and performance optimization.
I'm driven by the challenge of solving complex problems and creating elegant, efficient solutions. My goal is to deliver high-quality code that not only meets but exceeds client expectations, always keeping an eye on the latest industry trends and best practices.
Core Competencies
Laravel Ecosystem
Expert in Laravel and its ecosystem
Testing
Proficient in unit, integration, and feature testing
Scalable Architecture
Designing for growth and scalability
Database Optimization
Efficient data management and queries
Cloud Solutions
Deploying and managing cloud infrastructures
Security Best Practices
Implementing robust security measures
Skills & Expertise
Featured Projects
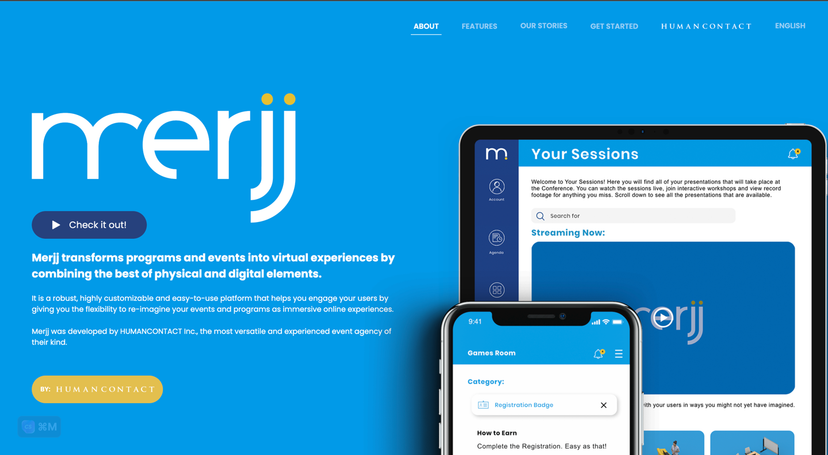





Code Showcase
This section highlights some of the solutions I've developed, challenges I've tackled, and code snippets showcasing my approach to solving real-world problems. These examples reflect my experience with Laravel, Next.js, Tailwind CSS, and API integrations.
Implemented eager loading with `with()` and chunk processing with `lazy()` to optimize the query.
use App\Models\Post;
$posts = Post::with('comments')->lazy(100);
foreach ($posts as $post) {
echo $post->title . ' (' . $post->comments->count() . ' comments)';
}
Outcome: Reduced query execution time by 40% and memory usage by 30%.
Implemented caching middleware using Next.js Edge Middleware and Redis to cache API responses.
import { NextRequest, NextResponse } from 'next/server';
import Redis from 'ioredis';
const redis = new Redis();
export async function middleware(req: NextRequest) {
const cachedResponse = await redis.get(req.url);
if (cachedResponse) {
return new NextResponse(cachedResponse, { status: 200 });
}
const response = await fetch(req.url);
const data = await response.text();
await redis.set(req.url, data, 'EX', 3600); // Cache for 1 hour
return new NextResponse(data, { status: response.status });
}
Outcome: Reduced server load by 50% and improved API response times for repeated queries.
Built a dynamic form generator that reads JSON configurations and renders forms with validation.
import React, { useState } from 'react';
const formConfig = [
{ label: 'Name', type: 'text', name: 'name', required: true },
{ label: 'Email', type: 'email', name: 'email', required: true },
{ label: 'Age', type: 'number', name: 'age', required: false },
];
export default function DynamicForm() {
const [formData, setFormData] = useState({});
const handleChange = (e) => {
setFormData({ ...formData, [e.target.name]: e.target.value });
};
const handleSubmit = (e) => {
e.preventDefault();
console.log(formData);
};
return (
<form onSubmit={handleSubmit}>
{formConfig.map((field) => (
<div key={field.name}>
<label>{field.label}</label>
<input
type={field.type}
name={field.name}
required={field.required}
onChange={handleChange}
/>
</div>
))}
<button type="submit">Submit</button>
</form>
);
}
Outcome: Reduced development time for form-based features by 70% and improved code reusability.
Utilized Laravel Sanctum to create a secure token-based authentication system.
// Sanctum setup in Laravel
php artisan require laravel/sanctum
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
php artisan migrate
// Enable SPA Authentication in middleware
use Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful;
protected $middlewareGroups = [
'web' => [
EnsureFrontendRequestsAreStateful::class,
],
];
Outcome: Improved security and simplified authentication process for single-page applications.
Passion Project
Sempre Studios is a digital agency I founded, specializing in building custom websites, landing pages, and web applications. We focus on helping small businesses in our area transition to the digital landscape without breaking the bank.
Our Expertise
- Custom Web Development
- Landing Page Design
- Web Application Development
- SEO Optimization
- Content Creation